Factory Method Design Pattern, UML diagram, Java Example
Definition
Define an interface for creating an object, but let subclasses decide which class to instantiate. Factory Method lets a class defer instantiation to subclasses.
Class Diagram
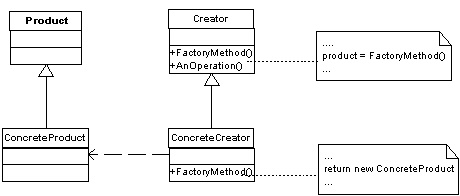
Factory Method pattern is a simplified version of Abstract Factory pattern.
Factory Method pattern is responsible of creating products that belong to one family, while Abstract Factory pattern deals with multiple families of products.
The official GoF Factory Method UML diagram (above) can be represented as in diagram below which uses the same terminology as Abstract Factory UML diagram:
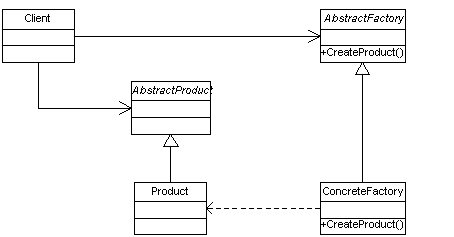
Participants
-
Product
- defines the interface of objects the factory method creates.
-
ConcreteProduct
- implements the Product interface.
-
Creator
- declares the factory method, which returns an object of type Product. Creator may also define a default implementation of the factory method that returns a default ConcreteProduct object.
- may call the factory method to create a Product object.
-
ConcreteCreator
- overrides the factory method to return an instance of a ConcreteProduct
Benefits
- Eliminates the needs to bind application classes into your code. The code deals woth interfaces so it can work with any classes that implement a certain interface.
- Enables the subclasses to provide an extended version of an object, because creating an object inside a class is more flexible than creating an object directly in the client.
Usage
- When a class cannot anticipate the class of objects it must create.
- When a class wants its subclasses to specify the objects it creates.
- Classes delegate responsability to one of several helper subclasses, and you want to localize the knowledge of which helper subclasses is the delegate.